Sequence Types
- Sequences allow you to store multiple values in an organized and efficient way.
- There are seven sequence types: strings, Unicode strings, lists, tuples, bytearrays, buffers, and xrange objects.
- Nothing to worry about looking at this long list; you will get to know it gradually.
Python Strings
- Strings are a sequence of characters.
- Let us see some examples of String: "My name is Rahul", "Rahul", "Go home". All these are examples of String.
- In Python, Strings are called str.
- There is a specific way of defining String in Python – it is defined within single quotes (‘) or double quotes (“), or even triple quotes (“‘).
Accessing String Elements
- Square brackets can be used to access elements of the string.
- Remember that the first character has index 0.
- Index refers to the position of a character in a string. In python index number starts from 0.
- Example:
1
a = "Hello, World!"
1
print(a[1])
- Will give an output e. Can you understand why?
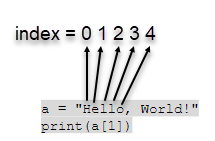
- Hope you got the answer to the previous question now!
String Slicing
- We can also call out a range of characters from the string using string slicing.
- Specify the start and end indexes separated by a colon to return a part of the string. Note that the character of the end index is not included.
- Suppose we want to print World from the “Hello World” string. We can do so as below:

Negative Indexing
-
If we have a long string and we want to pinpoint an item towards the end, we can also count backward from the end of the string, starting at the index number -1.
-
Printing ‘r’ from the string:
1
a = "Hello, World!"
1
print(a[-4])
-
Get the characters from position -5 to position -3, starting the count from the end of the string:
1
print(a[-5:-2])
-
Output: orl
String Concatenation
- String concatenation means adding strings together.
- Use the + character to add a variable to another variable:
- Example:
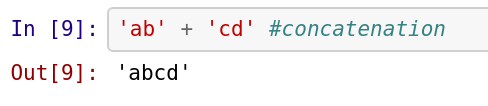
- Another example:
1 2 3 4
x = "Python is " y = "awesome" z = x + y print(z)
- Output: Python is awesome
String Concatenation: Add Space
- We can also add spaces between two strings
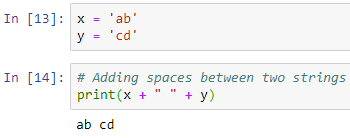
String Length
- To get the length of a string, use the len( ) function.
- Getting the length of the string a:
1 2
a = "Hello, World!" print(len(a))
- Output: 13
String Methods
- Python has a set of built-in methods that you can use on strings.
- Must learn: Learn about important string methods from the below cheatsheet: Python String Methods Cheatsheet
- Tip: If you cannot follow, run the code and figure out the difference.