Can you reshape an array?
YES!
- Using np.reshape() will change the shape of your array.
- Also, there won’t be any changes in the original data other than the shape.
- Caution: Whenever you use reshape() method, you need to make sure that the array you want to produce after reshaping needs to have the same number of elements as the original array.
- Suppose you have an array with 24 elements. If you want to reshape this array, you need to make sure that your new array also has a total of 24 elements. You can reshape this array into (8, 3), (6, 4), (12, 2), and so on, as all these will also have total of 24 elements (8
3 = 24, 6 4 = 24, 12 2 = 24). - Create an array with 24 elements in it.

- Use np.reshape() to change the shape of arrays as shown in the code snippet here.
- Array with shapes (8, 3), (6, 4) and (2, 12) have 24 elements in it.
- All of these three arrays have the same values that were present in the initial array ‘arr’.
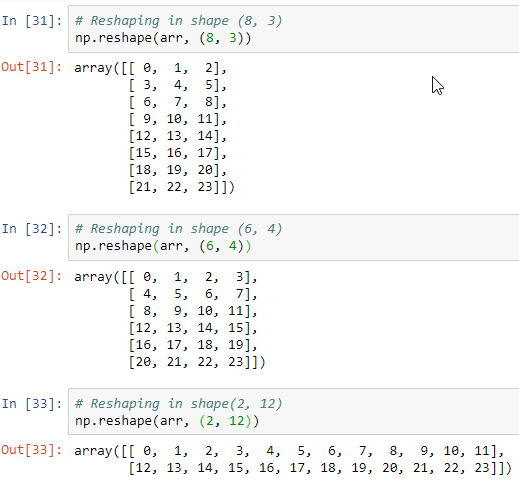
Reshaping a Python List
Using np.reshape() you can change the shape of a Python list but the returned object is of type numpy.ndarray, i.e., it becomes a NumPy array.
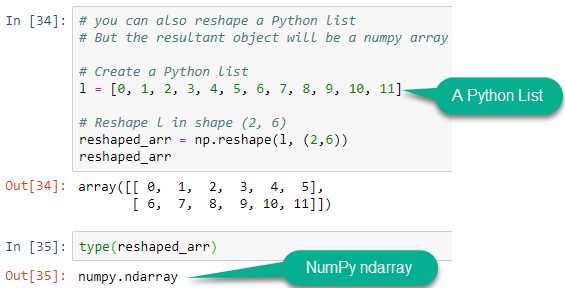
Using reshape() directly on Array
You can use reshape() method directly on the NumPy array:
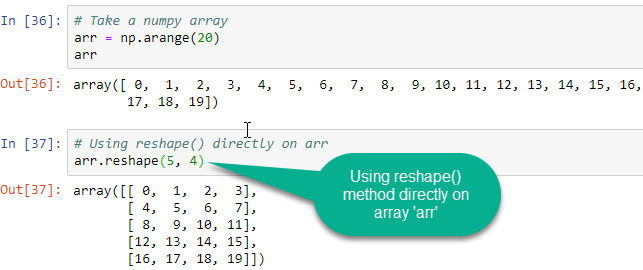
Caution! Using reshape() method on a Python list will throw an ‘AttributeError’.
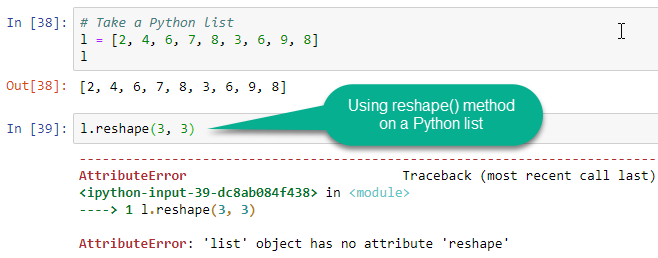